You have already properly identified the meaning of MVC in your question, Model-View-Controller, but essentially it is a design pattern that is used in programming and web development frameworks like you mentioned. You might also say it's an architectural pattern since it sort of forms the basis of how your application generally is pieced together.
If you have heard of the SOLID principle, MVC follows the "S" principle by separating responsibilities. The Model contains the data, the view renders the elements that interact with the user, and the controller ensures that the sequence of steps occurs correctly and pieces everything together.
Does that make it all crystal clear to you now? Probably not, you might be exactly where you were before with your understanding. Maybe a better question would be, What is the point of MVC, and how will it help me?
I think where this pattern excels is when you have rather complex systems, especially systems that you might reuse in different places. A common model for instance is a model representing the User
. With this model, you would probably be able to obtain all sorts of data, probably much from the database where it's stored, but you may also have other logic in the model that does calculations or has to run through logic before returning the value that is used. You can then load up this Model in a controller and send some of its data to a view that is presented to someone visiting your website. The controller itself would be rather simple, it would ask for the User Model and then would send any data obtained from this model to the view which would render the HTML and the data together.
Now imagine if you didn't use the MVC pattern, and instead, you had a single method in a class for each webpage on your website that ran through logic line-by-line. This method would have to do quite a bit of work, it would have to have logic in place to bring in the user's data (perhaps a unique database query), it would have to run any calculations, and it would need to somehow have logic in place for HTML and inserting any dynamic data in that HTML where it belongs. The old Ozzu which ran on PHPBB did exactly this sort of procedural programming, and it was often frustrating to work with. I always feared making certain changes because I was not sure how they might affect other areas. Everything was very coupled together.
So by using MVC, you will:
- Promote separation of responsibilities: Allows you to help make the code easier to maintain and extend since everything is decoupled.
- Support code reusability and scalability: Can easily change the view or model without affecting other components. Perhaps you want a view for when people visit the web page, but you also want a view for those accessing the data via an API. No problem, you can easily reuse the components.
- Maintain better organization of code: Always helpful, but even more so if you have a large or complex application.
Finally just a note about routing. Most MVC systems used with web applications will have some sort of routing system so that you can send (route) requests to a specific controller and method that will handle it. Laravel for instance sends everything through a routing system first so that you can do exactly this (if you want). The image below shows what the MVC model looks like with the routing portion included:
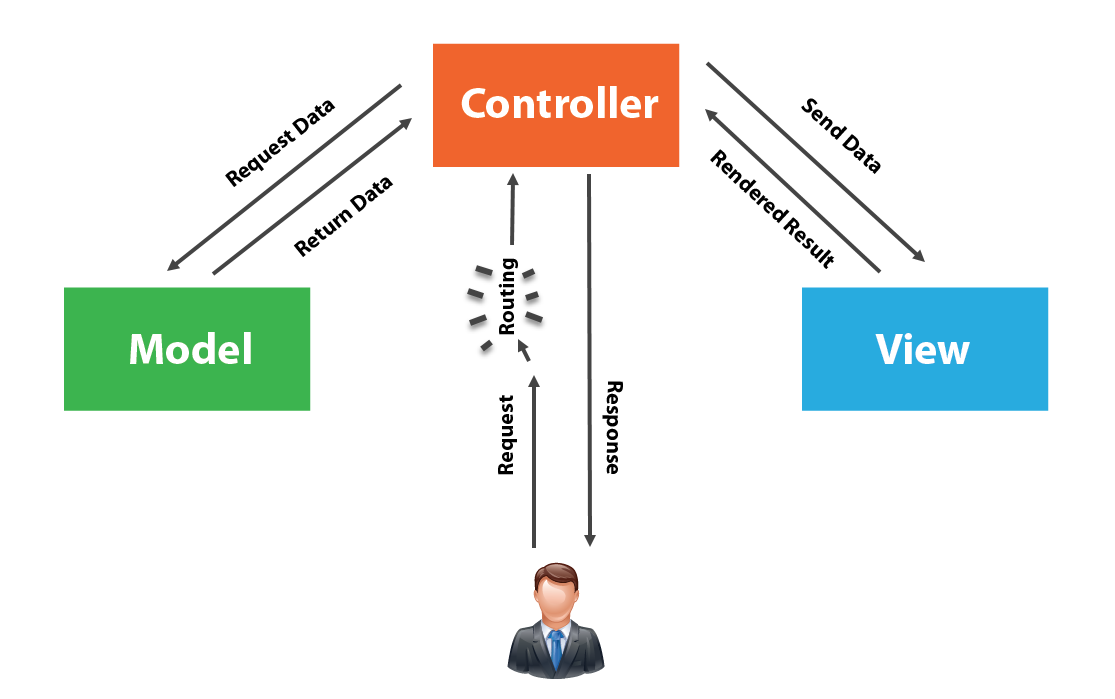
In a nutshell, it's just a way to figure out what controller/method is responsible for returning what the user asked for. From there the controller's method will get all of the data the view needs via models, get its rendered result from the view (HTML for a web page, JSON for an API response, etc), and then send it back to the user who requested it.